일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- word2vec
- deeplearning
- 가비아
- Selenium
- 딥러닝
- CBOW
- 예제중심HTML&자바스크립트&CSS
- 밑바닥부터시작하는딥러닝
- Crawling
- 크롤링
- 컴파일설치
- 소스설치
- 셀레니움
- 프로그램새내기를위한자바언어프로그래밍
- jupyter
- 수동설치
- MySQL
- Lamp
- 한빛아카데미
- attention
- 머신러닝
- 논문리뷰
- 밑바닥부터시작하는딥러닝2
- 생활코딩
- AndroidStudio를활용한안드로이드프로그래밍
- image
- 비지도학습
- aws
- 한빛미디어
- Apache
- Today
- Total
안녕, 세상!
6. 고급 위젯 본문
(1) 고급 위젯
① 아날로그시계, 디지털시계
시계 위젯은 시간을 표시하는 위젯으로 둘 다 다음과 같이 View 클래스에서 상속받습니다.
View 클래스 관련 속성을 사용할 수 있습니다.
java.lang.Object
ㄴ android.view.View
ㄴ android.widget.AnalogClock
ㄴ android.widget.TextView
ㄴ android.widget.DigitalClock
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
|
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<AnalogClock
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<DigitalClock
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center" />
</LinearLayout>
|
cs |
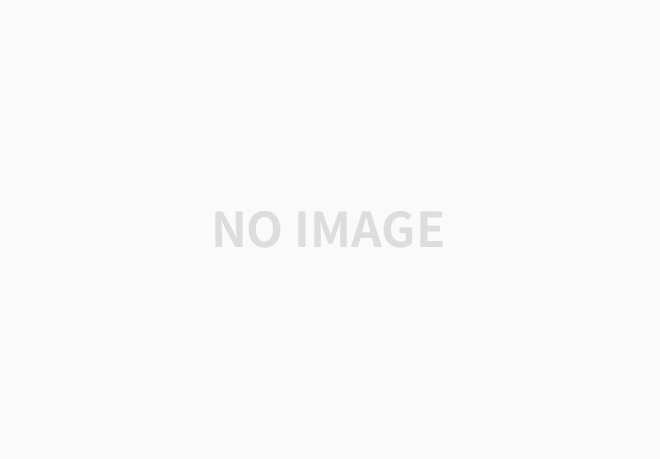
analogClock은 layout_width속성을 match_parent로 설정하면 자동으로 중앙으로 설정되고, DigitalClock은 layout_width속성을 match_parent로 설정해도 중앙으로 설정이 안 되므로 gravity속성으로 중앙에 오게 위치시켰습니다.
layout_width가 match_parent 일 경우는 layout_gravity가 적용이 안돼서 gravity속성으로 위치시켜야 합니다.
② Chronometer(크로노미터)
크로노미터는 타이머 형식의 위젯이며 일반적으로 시간을 측정할 때 많이 사용합니다.
java.lang.Object
ㄴ android.view.View
ㄴ android.widget.TextView
ㄴ android.widget.Chronometer
크로노미터의 java코드에서의 속성은 start(), stop() 등이 있습니다.
다음은 Chronometer를 이용한 코드 예제입니다.
acativity_main.xml
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
|
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content" >
<Chronometer
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:format="측정 : %s"
android:gravity="center"
android:id="@+id/chronometer1"
android:textSize="35dp" />
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:gravity="center">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/startbutton"
android:text="시작" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/stopbutton"
android:text="종료" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/resetbutton"
android:text="리셋" />
</LinearLayout>
</LinearLayout>
|
cs |
MainActivity.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
|
import androidx.appcompat.app.AppCompatActivity;
import android.graphics.Color;
import android.os.Bundle;
import android.os.SystemClock;
import android.view.View;
import android.widget.Button;
import android.widget.Chronometer;
public class MainActivity extends AppCompatActivity {
Chronometer chrono;
Button startbtn, stopbtn, resetbtn;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
startbtn = (Button) findViewById(R.id.startbutton);
stopbtn= (Button) findViewById(R.id.stopbutton);
resetbtn= (Button) findViewById(R.id.resetbutton);
chrono = (Chronometer) findViewById(R.id.chronometer1);
startbtn.setOnClickListener(new View.OnClickListener() {
public void onClick(View v){
chrono.setBase(SystemClock.elapsedRealtime());
chrono.start();
chrono.setTextColor(Color.RED);
}
});
stopbtn.setOnClickListener(new View.OnClickListener(){
public void onClick(View v){
chrono.stop();
chrono.setTextColor(Color.BLUE);
}
});
resetbtn.setOnClickListener(new View.OnClickListener(){
public void onClick(View v){
chrono.setBase(SystemClock.elapsedRealtime());
chrono.setTextColor(Color.BLACK);
}
});
}
}
|
cs |
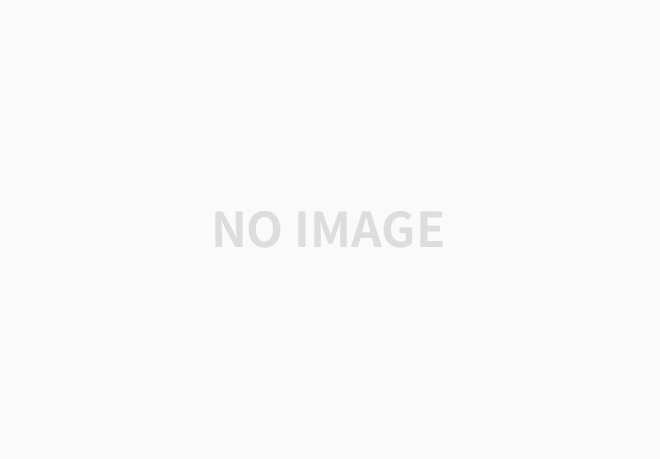
시작 버튼을 누르면 글씨 색상이 빨간색으로 변하고 시간이 흐르며 종료를 누르면 글씨 색상이 파란색으로 변하고 멈추고 리셋을 누르면 글씨가 검은색으로 변하고 다시 00:00으로 돌아옵니다.
setBase(SystemClock.elapsedRealtime()) : 크로노미터의 시간 값을 00:00으로 초기화 시킴
③ TimePicker, DatePicker, CalendarView
java.lang.Object
ㄴ android.view.View
ㄴ android.view.ViewGroup
ㄴ android.widget.TimePicker
ㄴ android.widget.DatePicker
ㄴ android.widget.CalendarView
TimePicker은 시간을 DatePicker은 날짜를 CalendarView는 달력을 표시하고 조절하는 기능을 합니다.
activity_main.xml
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TimePicker
android:timePickerMode="spinner"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<DatePicker
android:datePickerMode="spinner"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<CalendarView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:showWeekNumber="false"/>
</LinearLayout>
|
cs |
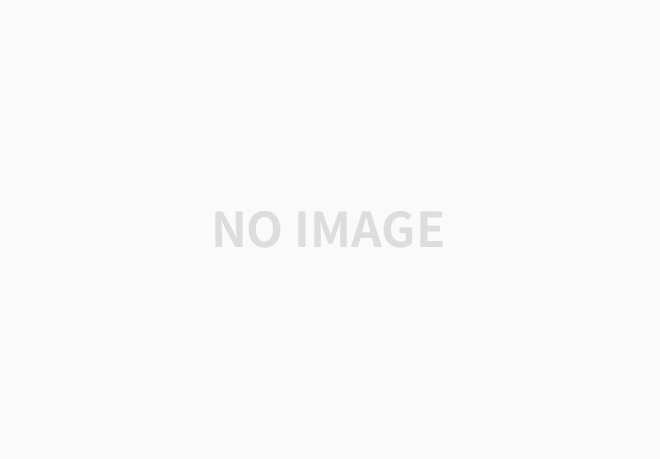
④ 자동완성텍스트뷰, 멀티 자동완성 텍스트뷰
자동완성 텍스트뷰는 AutoCompleteTextView이고 멀티 자동완성 텍스트뷰는 MultiAutoCompleteTextView입니다.
java.lang.object
ㄴ android.view.View
ㄴ android.widget.TextView
ㄴ android.widget.EditText
ㄴ android.widget.EditText.AutoCompleteTextView
ㄴ android.widget.EditText.MultiAutoCompleteTextView
자동완성텍스트뷰는 단어 1개가 자동 완성되고, 멀티 자동완성 텍스트뷰는 쉼표(,)로 구분하여 여러 개 단어가 자동 완성됩니다.
(자동완성 텍스트뷰는 쉼표를 한 단어로 인식해서 쉼표가 단어 구분 역할을 하지 못함)
activity_main.xml
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<AutoCompleteTextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/autoview"
android:completionHint="선택하세요."
android:completionThreshold="3"
android:hint="자동완성텍스트뷰" />
<MultiAutoCompleteTextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/multiview"
android:completionHint="선택하세요."
android:completionThreshold="3"
android:hint="멀티자동완성텍스트뷰"/>
</LinearLayout>
|
cs |
MainActivity.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
|
import android.os.Bundle;
import android.widget.ArrayAdapter;
import android.widget.AutoCompleteTextView;
import android.widget.Button;
import android.widget.Chronometer;
import android.widget.MultiAutoCompleteTextView;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
Chronometer chrono;
Button startbtn, stopbtn, resetbtn;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
String[] items = {"friends", "fringe", "friedchicken"};
AutoCompleteTextView auto = (AutoCompleteTextView) findViewById(R.id.autoview);
ArrayAdapter<String> adapter = new ArrayAdapter<String>(this, android.R.layout.simple_dropdown_item_1line,items);
auto.setAdapter(adapter);
MultiAutoCompleteTextView multi = (MultiAutoCompleteTextView) findViewById(R.id.multiview);
MultiAutoCompleteTextView.CommaTokenizer token = new MultiAutoCompleteTextView.CommaTokenizer();
multi.setTokenizer(token);
multi.setAdapter(adapter);
}
}
|
cs |
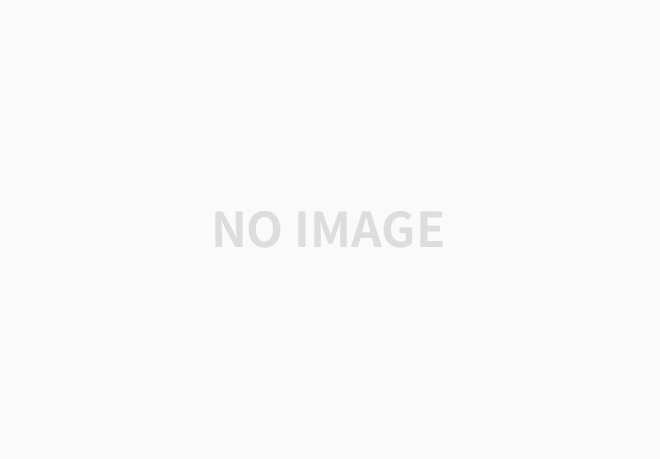
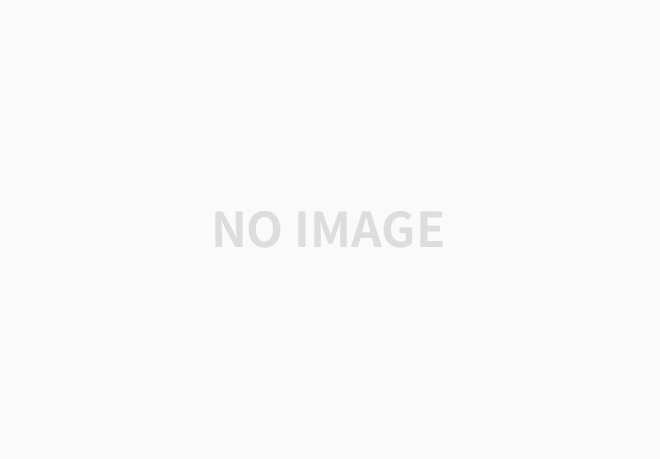
java 코드의 18번째 줄에서 items라는 배열에 자동 완성될 문자열을 정의합니다.
ArrayAdapter : 뷰와 데이터를 연결해주는 자료형
생성자의 두 번째 데이터인 simple_dropdown_item_1line 부분은 출력될 모양을 결정하는데 다양한 모양을 선택할 수 있음
21번째 줄의 코드로 뷰와 데이터를 연결해줌 ( .setAdapter() )
MultiAutoCompleteTextView.CommaTokenizer : 쉼표로 구분하기 위한 자료형
26번째 줄의 코드로 쉼표를 구분하게 적용 ( .setTokenizer() )
⑤ ProgressBar, SeekBar, RatingBar
이 위젯들은 진행상태를 표시하는 기능을 하는데 역할이나 용도가 조금씩 다릅니다.
(1) ProgressBar
작업의 진행 상태를 바(bar) 또는 원 형태로 제공합니다.
바 형태는 어느 정도 진행되었는지를 확인할 수 있지만, 원 형태는 현재 진행 중이라는 상태만 보여줍니다.
Xml 속성은 다음 속성들이 있습니다.
max : 범위 지정
progress : 시작 지점 지정
secondaryProgress : 두 번째 프로그레스 바 지정
(2) SeekBar
프로그레스 바의 하위 클래스로 프로그레스 바와 대부분 비슷하고 사용자가 터치로 임의 조절이 가능합니다.
(3) RatingBar
프로그레스 바의 하위 클래스이고 진행 상태롤 별 모양으로 표시합니다.
보통 선호도를 나타날 때 주로 사용합니다.
Xml 속성은 다음 속성들이 있습니다.
numStarts : 별의 개수를 정함
rating : 초깃값을 정함
stepSize : 한 번에 채워지는 개수를 정함
activity_main.xml
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
|
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<ProgressBar
android:layout_width="match_parent"
android:layout_height="wrap_content"
style="?android:attr/progressBarStyleHorizontal"
android:max="100"
android:progress="30"
android:secondaryProgress="50" />
<SeekBar
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:max="100"
android:progress="50" />
<RatingBar
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:numStars="5"
android:rating="2.5"
android:stepSize="0.5" />
</LinearLayout>
|
cs |
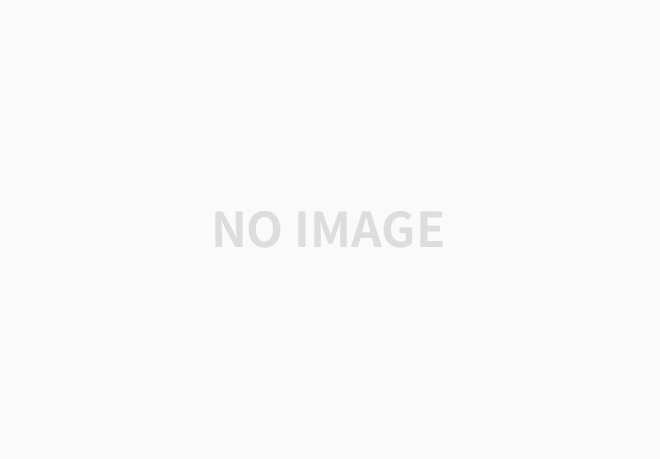
max속성과 Progress 속성은 비율로 생각하면 됩니다.
Seekbar과 RatingBar은 사용자가 직접 설정할 수 있습니다.
(2) View Container
ViewGroup클래스 하위의 위젯은 주로 다른 위젯을 담는 컨테이너 역할을 합니다.
① ScrollView
위젯이나 레이아웃이 화면에 넘칠 때 스크롤 뷰를 넣으면 스크롤 효과를 낼 수 있습니다.
ScrollView는 수직으로 스크롤하는 기능이며, 수평으로 스크롤하는 HorizontalScrollView는 따로 있습니다.
java.lang.Object
ㄴ android.view.View
ㄴ android.widget.ViewGroup
ㄴ android.widget.FrameLayout
ㄴ android.widget.ScrollView
스크롤 뷰는 단 하나의 위젯만 넣을 수 있습니다.
그래서 주로 스크롤 뷰 안에 리니어 레이아웃을 1개 넣고, 리니어 레이아웃 안에 여러 개의 위젯을 넣는 방법을 사용합니다.
activity_main.xml
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
|
<?xml version="1.0" encoding="utf-8"?>
<ScrollView xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<Button
android:layout_width="match_parent"
android:layout_height="120dp"
android:text="버튼 a" />
<Button
android:layout_width="match_parent"
android:layout_height="120dp"
android:text="버튼 b" />
<Button
android:layout_width="match_parent"
android:layout_height="120dp"
android:text="버튼 c" />
<Button
android:layout_width="match_parent"
android:layout_height="120dp"
android:text="버튼 d" />
<Button
android:layout_width="match_parent"
android:layout_height="120dp"
android:text="버튼 e" />
<Button
android:layout_width="match_parent"
android:layout_height="120dp"
android:text="버튼 f" />
<Button
android:layout_width="match_parent"
android:layout_height="120dp"
android:text="버튼 g" />
</LinearLayout>
</ScrollView>
|
cs |
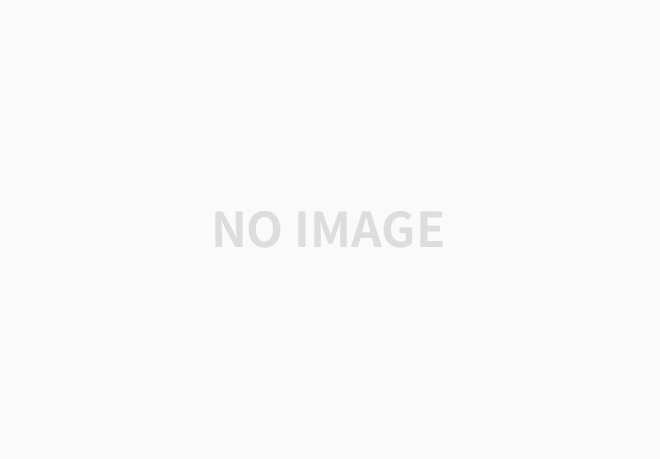
왼쪽에 스크롤바를 확인할 수 있으며 스크롤해서 아래의 값들을 확인할 수 있습니다.
ScrollBar는 수직 스크롤이므로 11번째 줄 layout 설정은 vertical로 해야 합니다.
수평 스크롤로 하고 싶으면 위의 코드에서 2, 44번째 줄을 HorizontalScrollView로 바꾸고 11번째 줄을 horizontal로 바꾸면 됩니다.
② SlidingDrawer
서람처럼 열어서 보여주거나 닫아서 감추는 위젯입니다.
java.lang.Object
ㄴ android.view.View
ㄴ android.widget.ViewGroup
ㄴ android.widget.SlidingDrawer
형식은 다음과 같습니다.
<SlidingDrawer
android:content="@+id/contentid"
android:handle="@+id/handleid" >
<Button
android:id="@+id/handleid" />
<LinearLayout
android:id="@+id/contentid" >
....서랍내부....
</LinearLayout>
</SlidingDrawer>
SlidingDrawer의 handle역할을 할 위젯의 id가 handle의 id와 동일해야 하고, content역할을 할 레이아웃의 id가 content의 id와 동일해야 합니다.
activity_main.xml
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
|
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="여기는 서랍 밖" />
<SlidingDrawer
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:content="@+id/contentid"
android:handle="@+id/handleid" >
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/handleid"
android:text="서랍" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:id="@+id/contentid"
android:background="#55FF0000"
android:gravity="center" >
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="여기는 서랍 안" />
</LinearLayout>
</SlidingDrawer>
</LinearLayout>
|
cs |
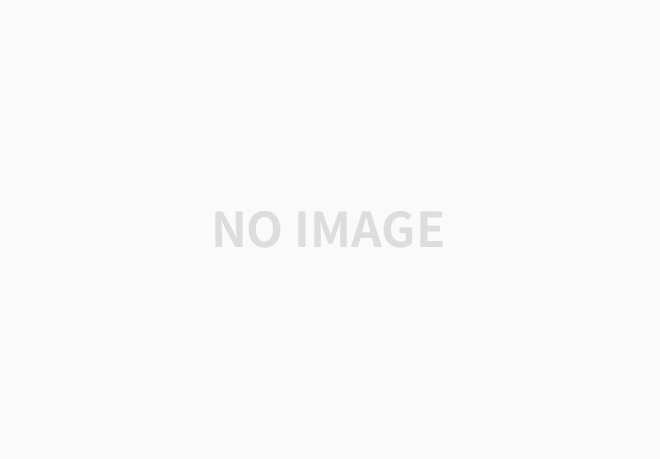
서랍 버튼을 누르면 위와 같이 서랍 안의 레이아웃이 서랍처럼 올라옵니다.
③ ViewFlipper
뷰 플리퍼는 안에 여러 개의 위젯을 배치하고 필요에 따라 화면을 왼쪽이나 오른쪽으로 밀어서 하나의 위젯씩 화면에 보여주는 방식의 뷰 컨테이너입니다.
java.lang.Object
ㄴ android.view.View
ㄴ android.widget.FrameLayout
ㄴ android.widget.ViewAnimator
ㄴ android.widget.ViewFlipper
Xml형식은 다음과 같습니다.
<LinearLayout>
<LinearLayout>
// 왼쪽/오른쪽으로 전환할 버튼 또는 이미지 뷰
</LinearLayout>
<ViewFlipper>
....한 번에 하나씩 보여줄 위젯을 이곳에 넣음
</ViewFlipper>
</LinearLayout>
다음은 ViewFlipper의 예제로 '이전 화면' 버튼을 누르면 이전 설정 레이아웃이 나오고 '다음 화면'버튼을 누르면 다음 설정 레이아웃이 나오는 코드입니다.
activity_main.xml
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
|
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:gravity="center">
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/prevbtn"
android:text="이전화면" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/nextbtn"
android:text="다음화면" />
</LinearLayout>
<ViewFlipper
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/viewf" >
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#FF0000" >
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#00FF00" >
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#0000FF" >
</LinearLayout>
</ViewFlipper>
</LinearLayout>
|
cs |
MainActivity.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
|
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.ViewFlipper;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
Button prevbtn, nextbtn;
ViewFlipper flipsample;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
prevbtn = (Button) findViewById(R.id.prevbtn);
nextbtn= (Button) findViewById(R.id.nextbtn);
flipsample = (ViewFlipper) findViewById(R.id.viewf);
prevbtn.setOnClickListener(new View.OnClickListener(){
public void onClick(View v){
flipsample.showPrevious();
}
});
nextbtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
flipsample.showNext();
}
});
}
}
|
cs |
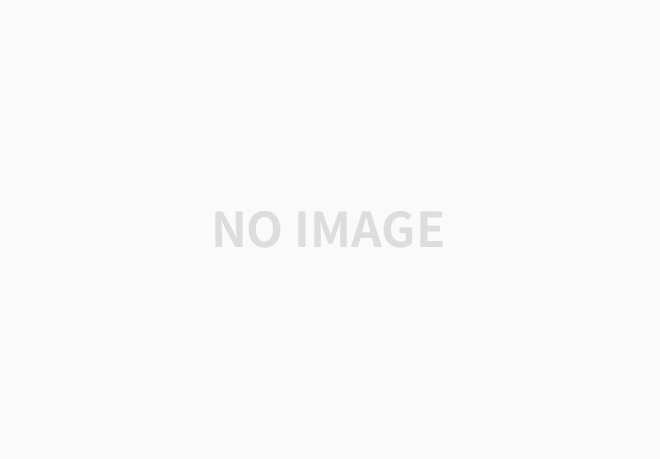
'다음 화면'버튼을 누르면 빨간색 화면이 나오고 '이전 화면'버튼을 누르면 초록색 화면이 나옵니다.
뷰 플립퍼 변수.showPrevious(); : 뷰 플리퍼에서 설정한 이전 위젯을 보여줌
뷰 플립퍼 변수.showNext();
④ TabHost
탭 호스트는 기존 화면이 넘어가고 다음 화면이 나오는 뷰 플리퍼와 달리 여러 탭을 두고 각 탭을 클릭할 때마다 해당 화면이 나오도록 설정한 뷰 컨테이너입니다.
웹브라우저의 상단 탭을 생각하면 됩니다.
java.lang.Object
ㄴ android.view.View
ㄴ android.widget.ViewGroup
ㄴ android.widget.FrameLayout
ㄴ android.widget.TabHost
Xml코드의 형식은 다음과 같습니다.
<TabHost
android:id="@android:id/tabhost">
<LinearLayout>
<TabWidget
android:id="@android:id/tabs" />
<FrameLayout
android:id="@android:id/tabcontent">
....이곳에 각 탭 스펙에 대응할 탭 화면(레이아웃)을 넣음
</FrameLayout>
</LinearLayout>
</TabHost>
TabHost의 id는 반드시 tabhost라고 해야 하고 <TabWidget>의 id는 반드시 "tabs"라고 해야 하고, <FrameLayout>의 id도 반드시 "tabcontent"라고 해야 합니다.
java코드 형식은 다음과 같습니다.
TabHost tabHost = getTabHost(); // 탭 호스트 변수 생성
// 탭 스펙 생성
TabSpec tabSpec1 = tabhost.newTabSpec("TAG1").setIndicator("탭에 출력될 글자");
tabSpec1.setContent(R.id.tabid); // 탭 스펙을 탭과 연결.
// tabid는 xml코드의 <FrameLayout>안에 있는 레이아웃 혹은 위젯의 id
tabHost.addTab(tabSpec1); // 탭을 탭 호스트에 부착
다음은 Tabhost를 활용한 예제입니다.
activity_main.xml
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
|
<?xml version="1.0" encoding="utf-8"?>
<TabHost xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:id="@android:id/tabhost">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<TabWidget
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@android:id/tabs">
</TabWidget>
<FrameLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@android:id/tabcontent">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:id="@+id/tabA"
android:background="#FF0000" >
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:id="@+id/tabB"
android:background="#00FF00" >
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:id="@+id/tabC"
android:background="#0000FF" >
</LinearLayout>
</FrameLayout>
</LinearLayout>
</TabHost>
|
cs |
MainActivity.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
|
import android.app.TabActivity;
import android.os.Bundle;
import android.widget.TabHost;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends TabActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
TabHost tabHost = getTabHost();
TabHost.TabSpec tabSpecA = tabHost.newTabSpec("A").setIndicator("Apple");
tabSpecA.setContent(R.id.tabA);
tabHost.addTab(tabSpecA);
TabHost.TabSpec tabSpecB = tabHost.newTabSpec("B").setIndicator("Banana");
tabSpecB.setContent(R.id.tabB);
tabHost.addTab(tabSpecB);
TabHost.TabSpec tabSpecC = tabHost.newTabSpec("C").setIndicator("Cock");
tabSpecC.setContent(R.id.tabC);
tabHost.addTab(tabSpecC);
tabHost.setCurrentTab(0);
}
}
|
cs |
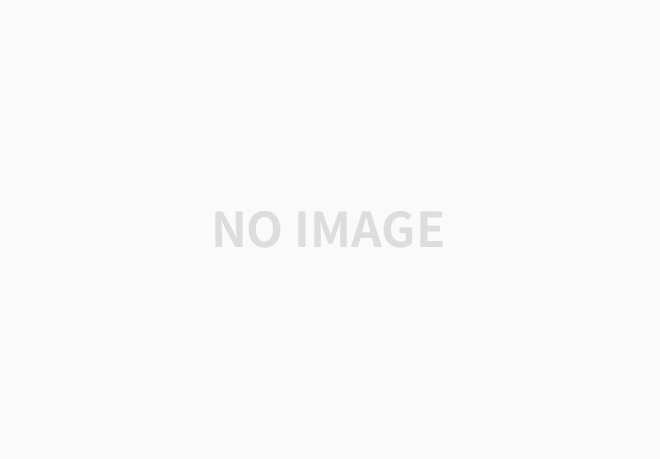
java코드
7번째 줄 extends TabActivity로 바꿔야 합니다.
탭 호스트 변수.setCurrnetTab(); : 초기에 어떤 탭을 보여줄지 설정
위의 예제에서는 '0'인 경우에는 'Apple'탭을, '1'인 경우에는 'Banana'탭을, '2'인 경우에는 'Cock'탭을 초기에 보여줌
⑤ 웹뷰(WebView)
웹뷰는 사용자가 웹브라우저 기능을 앱 안에 직접 포함할 수 있는 위젯입니다.
실제 웹브라우저처럼 만들려면 많은 작업이 필요하지만 여기선 간단하게 만들어 보겠습니다.
웹뷰 이용 시 기본 URL 주소는 보안 주소인 https:// 로 이루어진 주소를 사용해야 됩니다.
java.lang.Object
ㄴ android.view.Veiw
ㄴ android.widget.ViewGroup
ㄴ android.widget.AbsoluteLayout
ㄴ android.webkit.WebView
[app] -> [res] -> [drawable]에 이미지를 넣어서 앱의 런쳐 모양도 바꿔보겠습니다.
[app] -> [res] -> [drawable]에 이미지를 넣는 방법
우선 이미지 파일을 png형태로 저장을 하고 이미지 파일을 복사합니다.
그 후 [drawable]에 오른쪽 마우스 클릭 후 [paste]를 누릅니다.
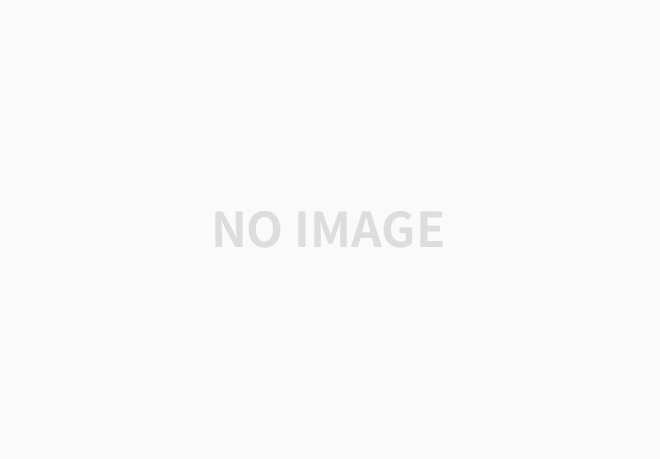
drawble을 선택 하고 [ok]를 누릅니다.
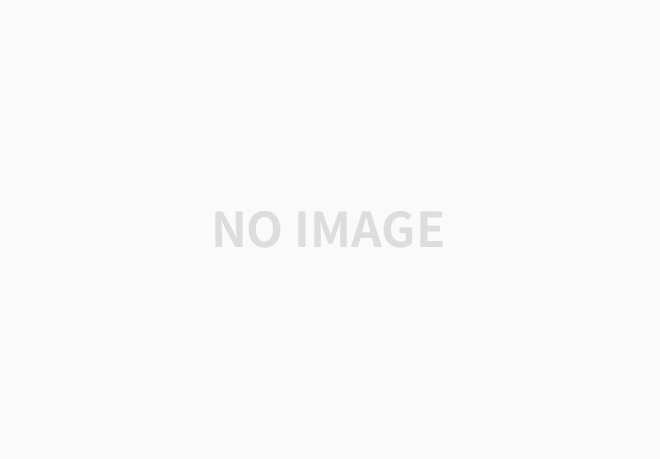
해당 그림파일의 이름을 정하고 [ok]를 누릅니다.
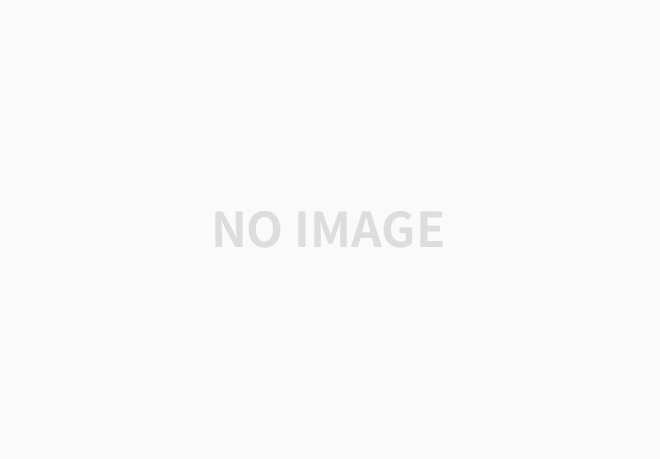
저는 다음과 같은 이미지를 ieicon.png라고 저장했습니다.
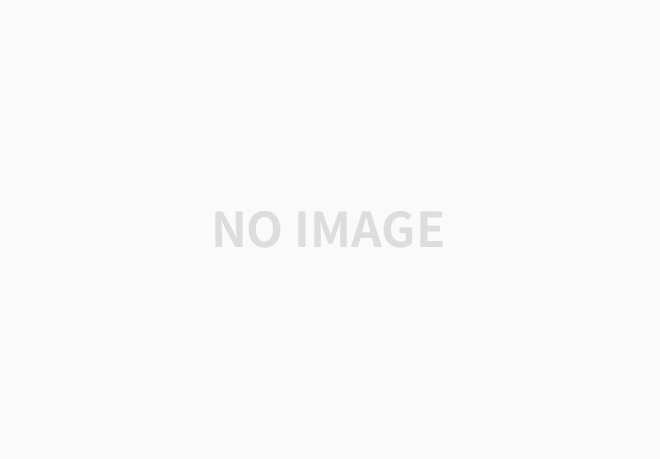
이미지를 우선 [drawable]에 넣었고 이제 xml부터 코드를 대입하겠습니다.
activity_main.xml
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
|
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical" >
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<EditText
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/edtURL"
android:layout_weight="1"
android:singleLine="true"/>
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/btngo"
android:text="이동" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/btnback"
android:text="이전" />
</LinearLayout>
<WebView
android:id="@+id/webView1"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</LinearLayout>
|
cs |
AndroidManifest.xml
프로젝트의 전반적인 환경을 설정하기 위해서 AndroidManifest.xml을 만져줘야 합니다.
Project Tree에서 [app] -> [manifests] -> [AndroidManifest.xml]을 더블클릭해서 열어줍니다.
코드는 다음과 같이 설정합니다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.application3">
<uses-permission android:name="android.permission.INTERNET" />
<application
android:allowBackup="true"
android:icon="@drawable/ieicon"
android:label="나의 웹브라우저앱"
android:theme="@style/AppTheme"
android:usesCleartextTraffic="true" >
<activity
android:name=".MainActivity"
android:label="간단 웹브라우저">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
|
cs |
4 : 프로젝트가 인터넷 사용을 허가한다는 의미
8 : 앱 런쳐의 아이콘을 설정함 (위에서 [drawable]에 넣은 이미지 불러서 사용)
9 : 앱의 레이블을 지정함 ([앱 정보] 창에 보이는 이름)
11 : http://로 된 주소도 불러올 수 있게 설정
12~20 : Activity에 대한 정보를 설정함. (여기서는 1개를 사용했지만, 여러 개의 액티비티를 사용할 수 도 있음)
14 : 앱 런쳐의 이름 (실행될 때 보이는 이름)
위와 같이 설정하면 다음과 같이 바뀐 것을 확인할 수 있습니다.
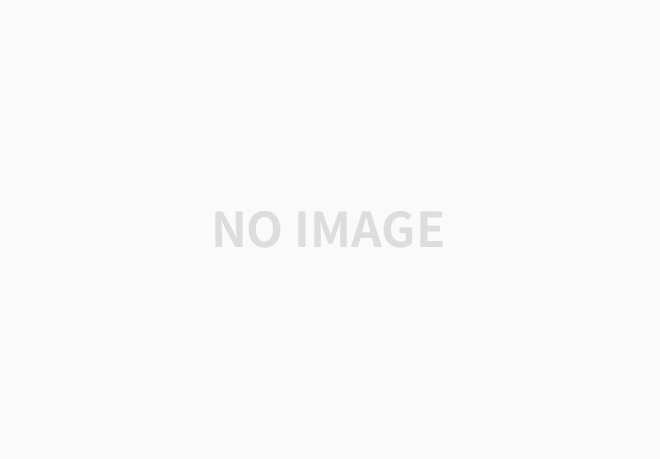
이제 Activity_main.xml에서 설정한 버튼 및 웹뷰가 잘 동작시키게 하기 위해서 java코드를 설정합니다.
MainActivity.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
|
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.webkit.WebSettings;
import android.webkit.WebView;
import android.webkit.WebViewClient;
import android.widget.Button;
import android.widget.EditText;
public class MainActivity extends AppCompatActivity {
EditText edturl;
Button btnGo, btnBack;
WebView wv;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
edturl = (EditText) findViewById(R.id.edtURL);
btnGo = (Button) findViewById(R.id.btngo);
btnBack = (Button) findViewById(R.id.btnback);
wv = (WebView) findViewById(R.id.webView1);
wv.setWebViewClient(new MyWebViewClient());
WebSettings webset = wv.getSettings();
webset.setBuiltInZoomControls(true);
btnGo.setOnClickListener(new View.OnClickListener(){
public void onClick(View v){
wv.loadUrl(edturl.getText().toString());
}
});
btnBack.setOnClickListener(new View.OnClickListener(){
public void onClick(View v){
wv.goBack();
}
});
}
class MyWebViewClient extends WebViewClient {
@Override
public boolean shouldOverrideUrlLoading(WebView view, String url) {
return super.shouldOverrideUrlLoading(view, url);
}
}
}
|
cs |
28~29 : WebSettings 클래스를 이용하여 줌 버튼 컨트롤이 화면에 보이게 함
43 : WebViewClient를 상속받는 MyWebViewClient 클래스를 만듦
33 : 에디트텍스트에 입력한 URL 웹페이지가 웹뷰에 나오게 함
MyWebViewClient를 만들 때 WebViewClient 클래스에서 shouldOverrideUrlLoading을 상속받으려는 과정에서 다음과 같이 하면 편리하게 자동으로 상속받을 수 있습니다.
클래스 명인 MyWebViewClient에 커서를 둔 상태로 [Code] -> [Override Methods]를 선택합니다.
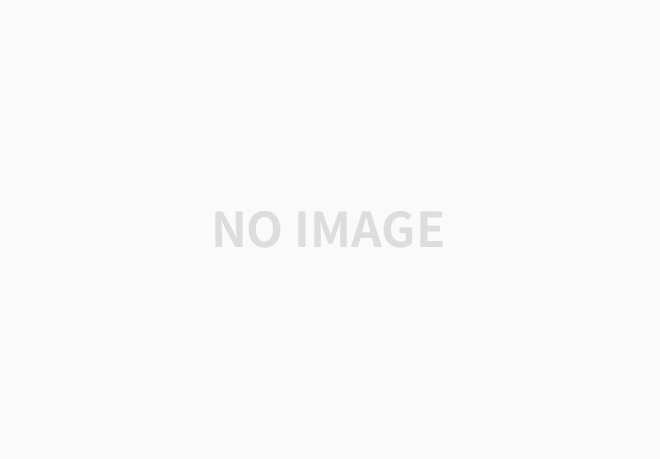
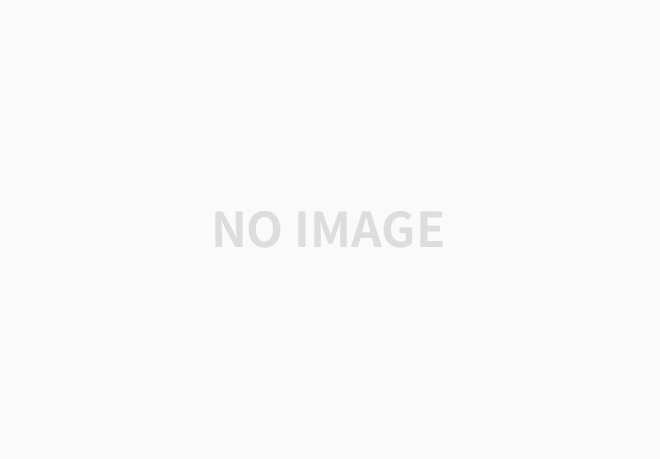
두 번째에 있는 shouldOverridingUrlLoading()을 선택하고 [ok] 버튼을 누르면 자동으로 코드가 생깁니다.
위 세 개의 코드를 모두 설정하고 동작시키면 다음과 같이 동작이 잘 됩니다.
URL을 입력하고 '이동'버튼을 누르면 잘 나옵니다.
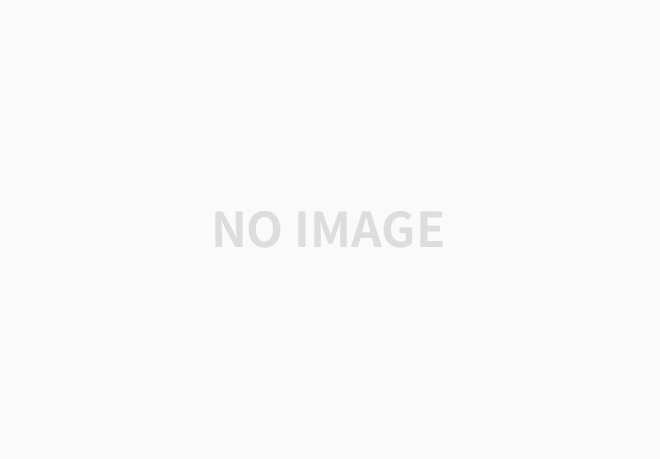
위에서 말했듯이 웹뷰는 기본적으로 https:// 로 이뤄진 주소를 사용해야 합니다.
'It공부 > 안드로이드' 카테고리의 다른 글
8. 파일 처리 (0) | 2020.08.24 |
---|---|
7.메뉴와 대화상자 (0) | 2020.08.23 |
5. 레이아웃 (0) | 2020.08.21 |
4. 기본 위젯 (0) | 2020.08.20 |
3. Java 문법 (0) | 2020.08.17 |